class: center, middle, inverse, title-slide .title[ # Code and Data Slides Template ] .author[ ### S. Mason Garrison ] --- # Simple Code Chunk Here's a simple R code chunk: ``` r x <- 3 * 4 y <- 10 - 2 z <- x + y print(z) ``` ``` ## [1] 20 ``` --- # Code with Output This code chunk will show both the code and its output: ``` r # Create a vector numbers <- c(1, 2, 3, 4, 5) # Calculate mean and standard deviation mean_value <- mean(numbers) sd_value <- sd(numbers) # Print results cat("Mean:", mean_value, "\n") ``` ``` ## Mean: 3 ``` ``` r cat("Standard Deviation:", sd_value) ``` ``` ## Standard Deviation: 1.581139 ``` --- # Code with Highlighted Lines In this example, we'll highlight important lines: ``` r # Load the tidyverse package library(tidyverse) # Create a simple dataframe df <- tibble( x = 1:5, y = c(2, 4, 6, 8, 10) ) # Plot the data ggplot(df, aes(x, y)) + geom_point() + geom_line() ``` 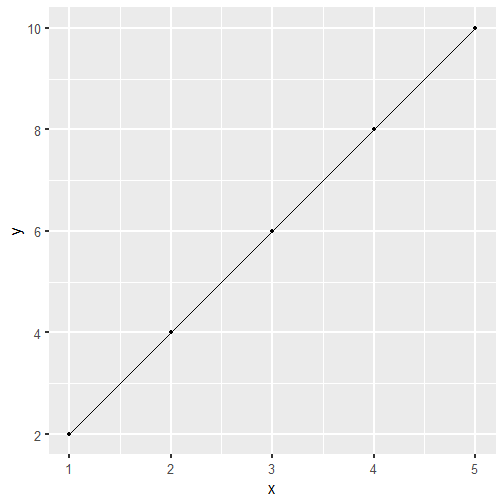<!-- --> --- # Data Visualization Code Here's an example of creating a data visualization with code: ``` r conflicts_prefer(palmerpenguins::penguins,.quiet = TRUE) ggplot(penguins, aes(x = flipper_length_mm, y = body_mass_g, color = species)) + geom_point(alpha = 0.7,na.rm=TRUE ) + labs(title = "Penguin Size", x = "Flipper Length (mm)", y = "Body Mass (g)", color = "Species") + theme_minimal() ``` 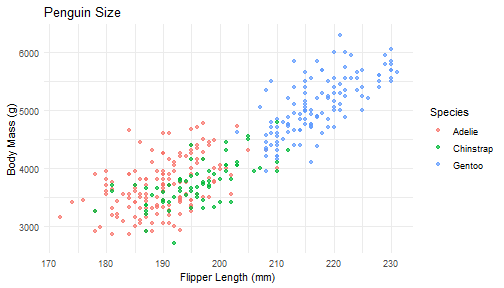<!-- --> --- # Data Manipulation This slide demonstrates data manipulation with dplyr: ``` r penguins %>% group_by(species, island) %>% summarize( mean_body_mass = mean(body_mass_g, na.rm = TRUE), mean_flipper_length = mean(flipper_length_mm, na.rm = TRUE) ) %>% arrange(desc(mean_body_mass)) ``` ``` ## # A tibble: 5 × 4 ## # Groups: species [3] ## species island mean_body_mass mean_flipper_length ## <fct> <fct> <dbl> <dbl> ## 1 Gentoo Biscoe 5076. 217. ## 2 Chinstrap Dream 3733. 196. ## 3 Adelie Biscoe 3710. 189. ## 4 Adelie Torgersen 3706. 191. ## 5 Adelie Dream 3688. 190. ``` --- # Interactive Code Output This slide shows how to create an interactive table: ``` r library(DT) penguins %>% select(species, island, body_mass_g, flipper_length_mm) %>% datatable() ```
--- # Code and Plot Side by Side .pull-left[ ``` r ggplot(mtcars, aes(x = wt, y = mpg)) + geom_point() + geom_smooth(method = "lm", se = FALSE,formula='y~x') + labs( title = "Car Weight vs. Miles Per Gallon", x = "Weight (1000 lbs)", y = "Miles Per Gallon" ) ``` ] .pull-right[ <img src="d00_code_files/figure-html/unnamed-chunk-1-1.png" width="100%" /> ] --- # Statistical Analysis This slide demonstrates a simple statistical test: ``` r # Perform a t-test t_test_result <- t.test(flipper_length_mm ~ sex, data = penguins) # Print the results print(t_test_result) ``` ``` ## ## Welch Two Sample t-test ## ## data: flipper_length_mm by sex ## t = -4.8079, df = 325.28, p-value = 2.336e-06 ## alternative hypothesis: true difference in means between group female and group male is not equal to 0 ## 95 percent confidence interval: ## -10.064811 -4.219821 ## sample estimates: ## mean in group female mean in group male ## 197.3636 204.5060 ``` --- ## Residuals Plot .pull-left[ ``` r depth_length_fit <- linear_reg() %>% set_engine("lm") %>% fit(bill_depth_mm ~ bill_length_mm, data = penguins) depth_length_fit_aug <- augment(depth_length_fit$fit) ggplot(depth_length_fit_aug, mapping = aes(x = .fitted, y = .resid)) + geom_point(alpha = 0.5) + geom_hline(yintercept = 0, color = "#bf2986ff", lty = "dashed") + labs(x = "Predicted Height", y = "Residuals") ``` ] .pull-right[ <img src="d00_code_files/figure-html/unnamed-chunk-2-1.png" width="100%" /> ] --- ## Model Output ``` r linear_reg() %>% set_engine("lm") %>% fit(bill_depth_mm ~ bill_length_mm, data = penguins) %>% tidy() ``` ``` ## # A tibble: 2 × 5 ## term estimate std.error statistic p.value ## <chr> <dbl> <dbl> <dbl> <dbl> ## 1 (Intercept) 20.9 0.844 24.7 4.72e-78 ## 2 bill_length_mm -0.0850 0.0191 -4.46 1.12e- 5 ``` --- ## Data Exploration .pull-left[ ``` r ggplot(penguins, aes(x = bill_depth_mm)) + geom_histogram(binwidth = 5) + labs(x = "Bill Depth (mm)", y = NULL) ``` ``` ## Warning: Removed 2 rows containing non-finite outside the scale range ## (`stat_bin()`). ``` ] .pull-right[ ``` ## Warning: Removed 2 rows containing non-finite outside the scale range ## (`stat_bin()`). ``` <img src="d00_code_files/figure-html/unnamed-chunk-3-1.png" width="100%" /> ]